QR Codes (Quick Response Codes) are perfect for visually encoding data. In fact, every camera device can read and decode them. That’s why they are often used for sharing links and other data with smartphone users. If you want to create an application that makes use of those QR codes, you should keep reading to find out how to decode and encode customizable QR codes from images and live webcam feed using Python!
Table of contents:
Install Required Packages
To get started, we first need to install some packages that are required to make the following codes work. If you haven’t installed pip yet, you can check out the tutorial here.
To install the packages, open your terminal (CMD/PowerShell on Windows) and type in the following command:
pip3 install opencv-python qrcode Image
Here’s why we need these packages:
opencv-python → Used for accessing images and the webcam, as well as decoding QR codes
qrcode → Used for encoding QR codes and saving them as an image
Image → Used by qrcode to save images
How To Create a QR Code
Let’s get into coding the encoder!
First, you need to create a new Python file with the .py extension. Of course, you can include this code in any already existing project.
Fortunately, encoding a QR code using Python is very easy and doesn’t require any further explanation. So here’s the code:
import qrcode
# This line sets the data the QR code should contain and encodes it:
qr = qrcode.make("https://coolplaydev.com")
# Save the QR code as a png file (can also be a jpg)
qr.save("qr.png")
Here’s the output of this particular code:
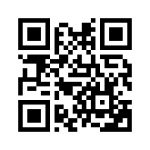
Changing Fill and Background Color
If you want a more customized QR code, you can use this code to change the fill color and background color of your QR code:
import qrcode
qr = qrcode.QRCode() # Instantiate a new QR code object
qr.add_data("https://coolplaydev.com") # Add the data to the QR Code
qr.make() # Generate the QR code
# In this line you can set the fill_color and back_color (You don't have to use both):
img = qr.make_image(fill_color="red", back_color="blue")
# Save the image as a png file (can also be a jpg)
img.save("qrFillColor.png")
This code generates the following QR code:
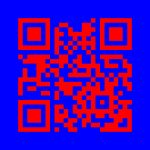
How To Decode QR Codes
Decoding a QR code isn’t really harder than encoding one.
Luckily, the following code doesn’t need any further explanation. Just copy it into your project:
import cv2
# Open the desired image (can also be a jpg)
img = cv2.imread("qrcode.png")
# Instantiate a new detector object
detector = cv2.QRCodeDetector()
# Decode the image
data = detector.detectAndDecode(img)
# Show the results
print(data[0])
Basically, this is the whole magic behind decoding QR codes from an image file.
Keep reading to find out how to decode QR codes from a live webcam feed!
How To Decode QR Codes From a Live Webcam Feed
Usually, we want to scan QR codes in real-time using a webcam. Luckily, OpenCV (Open Computer Vision) provides us with the perfect tools for accessing the webcam and decoding QR codes live.
Here’s the code:
import cv2
# Access the webcam
cap = cv2.VideoCapture(0)
# Instantiate a detector object
detector = cv2.QRCodeDetector()
# endless loop
while True:
# Read an image from the webcam
_, img = cap.read()
# Try to encode QR codes in the captured image
data, verts, _ = detector.detectAndDecode(img)
# Check if a QR code was detected
if verts is not None and data:
# print the decoded data
print("QR Code detected: ", data)
# Show the live webcam feed in a new window
cv2.imshow("QR Code Decoder", img)
# Press q to exit . . .
if cv2.waitKey(1) == ord("q"):
break
# Release the webcam and close the window
cap.release()
cv2.destroyAllWindows()
Conclusion
As you can see, coding a QR code encoder/decoder is very easy but can be useful in various projects.
Feel free to include the code snippets in your projects!
Thanks for reading!